How to send events
After inserting Finteza JavaScript Client, you can send website user action events to Finteza. To do this, add the following JavaScript code to the website page:
fz( "event", "{EVENT_NAME}" ); |
{EVENT_NAME} stands for a certain event name, for example, "Registration".
Check the code operation by running it through the browser console. If successful, you will see the corresponding entry in the Events website report of the Finteza panel.
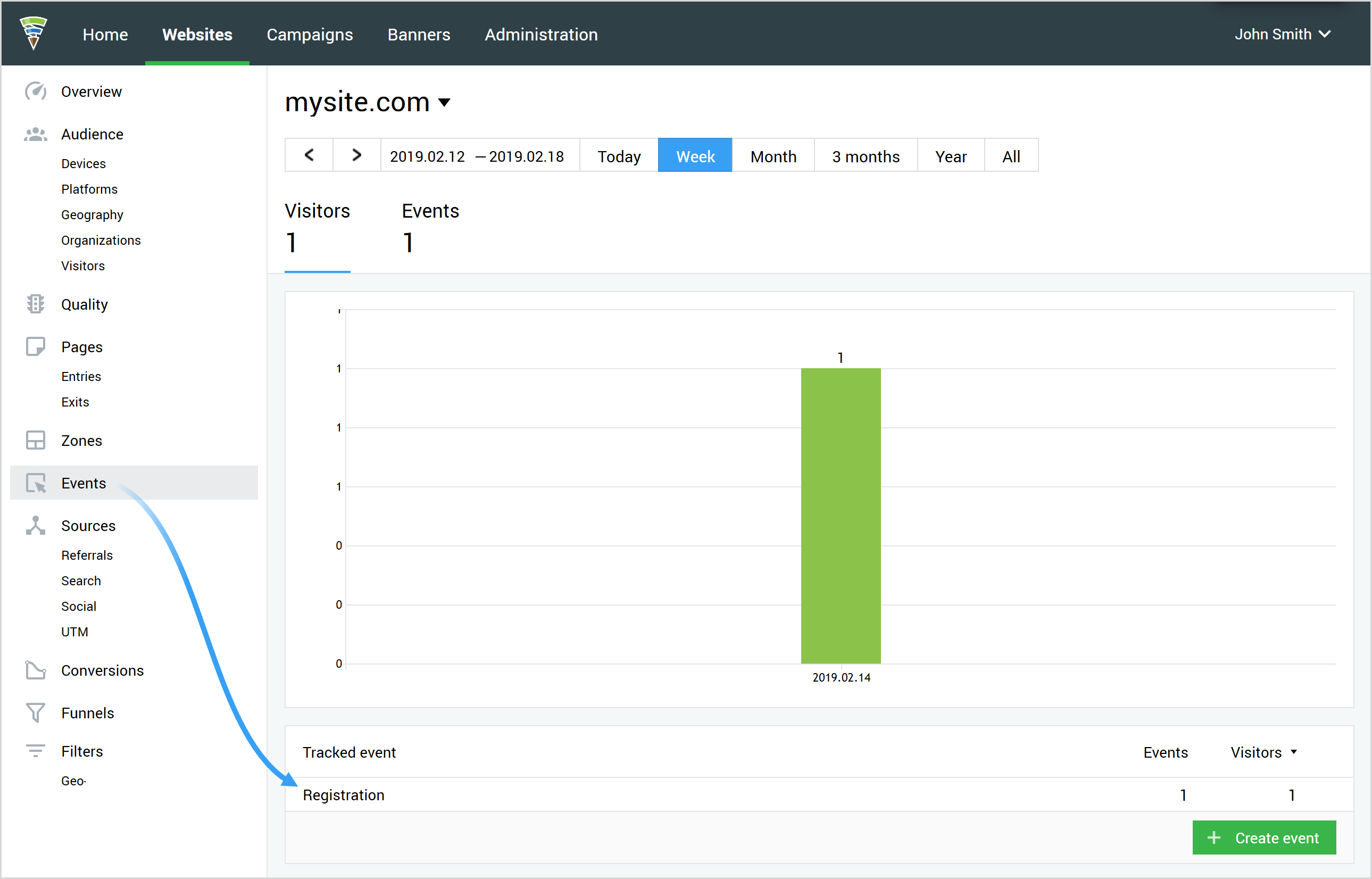
Additional data in the event parameters #
In events sent to Finteza, you can specify additional data as parameters, for example, a type of a purchased good, price, currency, etc.
fz( "event", {
|
Set the event name in {EVENT_NAME}. The following parameters are specified next:
Parameter |
Type |
Description |
---|---|---|
unit |
string |
Parameter measurement units, for example, USD, items, etc. The maximum length is 32 symbols. |
value |
number |
Parameter value. The maximum length is 64 symbols. |
Example:
fz( "event", {
|
Options for sending events from the web page #
The tracking code should be inserted into certain web page tags depending on a tracked event.
Sending an event when filling in the form:
<form action="" method="get" onsubmit="fz('event', 'Form+Order+Submit'); return true;">
|
Sending an event when clicking the button:
<form action="">
|
Sending an event by clicking on a link:
<a href="https://www.example.com/" onclick="fz('event', 'Click+Link'); return true;">www.example.com</a> |
Sending an event when loading a web page:
<body>
|
Auto handling of clicks by links #
Add the data-fz-event attributes to your website links and specify in them the names of events to be sent to Finteza when clicked. Finteza JavaScript Client automatically finds and handles clicking all such links on the web page.
<a data-fz-event="Click+Link" href="https://www.example.com/">www.example.com</a> |
This option can be used instead of adding the onclick attributes with the complete description of the fz() call. It is simpler and more convenient.
Similarly, you can pass additional 'unit' and 'value' parameters along with events. To do this, use the data-fz-unit and data-fz-value attributes:
<a data-fz-event="Click+Link" data-fz-unit="USD" data-fz-value="100" href="https://www.example.com/buy">Buy Product</a> |
For Google AMP Instant Articles, in which an individual tracking code version is used, an additional attribute naming requirement applies: they must start with the "data-vars" prefix. Therefore, you should add the data-vars-fz attributes to them to enable automatic processing of clicks on such links:
<a data-vars-fz="Click+Link" href="https://www.example.com/">www.example.com</a> |
To avoid confusion in naming, you can use data-vars-fz attributes everywhere. They are supported not only on Google AMP pages, but also on regular pages with the standard tracking code. |
Callback #
If you need to handle a sending event, set the callback function in the event code. Change the call second argument the following way:
fz( "event", {
|
It will be an object containing an event name and a link to the handler function.