Displaying ads
If your application displays ads, you can relocate the entire ad management to Finteza. Advertising campaigns on Android devices can be launched just in time and for all users at once. No application modifying, waiting for the publication of a new version on Google Play and updating of all devices is required.
In fact, you only need to define ad areas in your application, while the entire contents management, detailed statistics and conversions are available in Finteza.
Although advertisements are called banners in Finteza, they are not limited to static images and GIF animations. A banner is a container that may include a variety of contents types, including HTML5: landing pages, carousels, pop-ups, etc.
The FintezaBannerView class is responsible for downloading and displaying the banner contents in SDK.
Creating an advertisement zone in Finteza #
Before creating a banner in the application, configure an advertisement zone in Finteza. It allows you to manage the ad block contents.
Go to the Websites section of the Finteza panel, find your application and click "new zone":
- Set the name and allowed content formats. JPG, PNG, GIF and HTML are currently supported.
- Set the zone size if known in advance or select automatic zone scaling.
- Save the changes and copy the zone ID. It should be specified in the application.
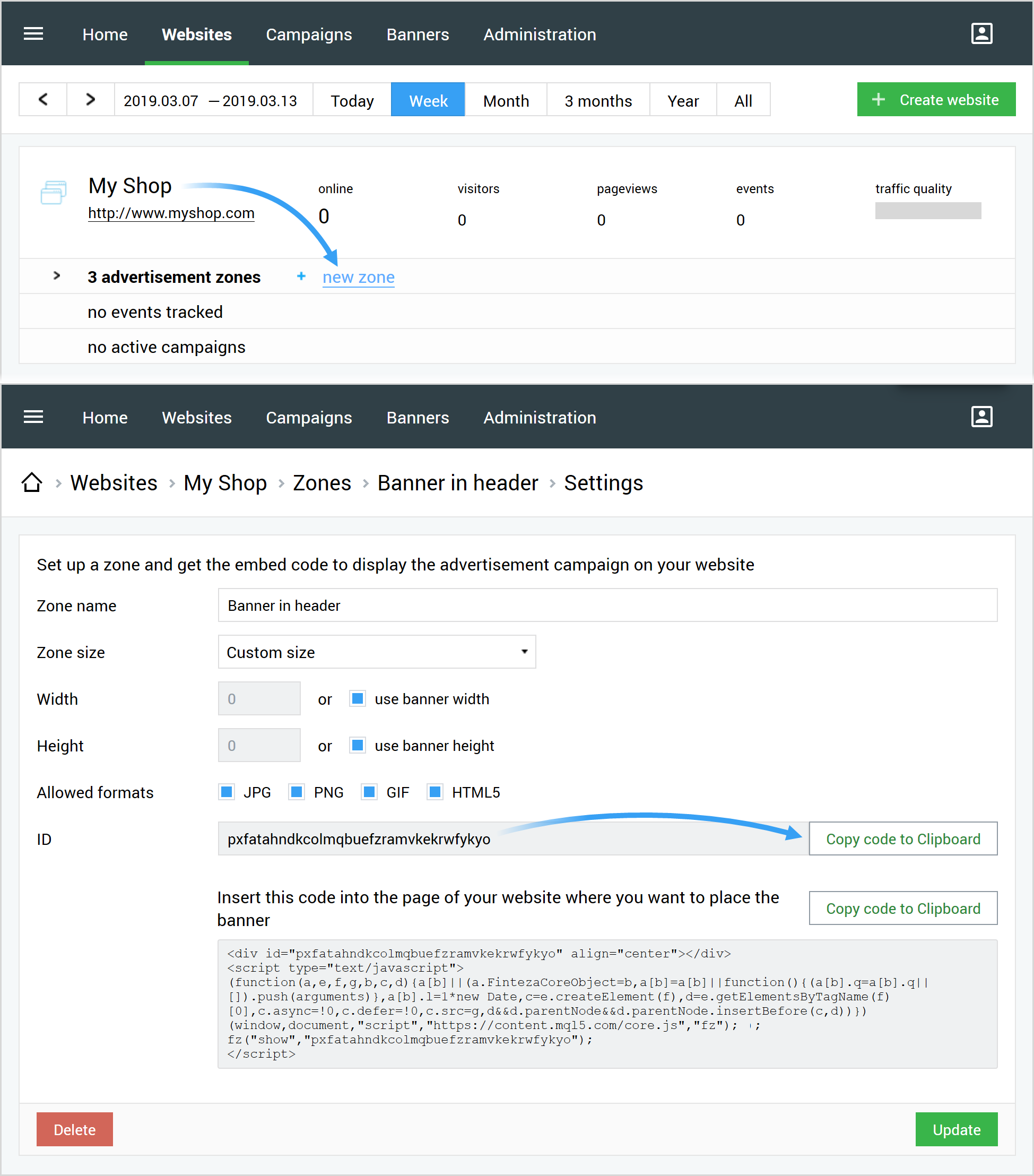
Creating a banner in the application #
You can create a banner by adding it to XML Layout or programmatically.
Create a layout object:
<net.metaquotes.finteza.banner.FintezaBannerView
|
In {WEBSITE_URL}, specify the application name or website domain the zone corresponds to. For {ZONE_ID}, indicate Finteza ad zone ID. These parameters are mandatory.
You can additionally specify the rotation time in seconds, in the optional parameter rotationTime. The default time is set to 10 minutes.
app:rotationTime="20" |
Use the following code to create a banner:
FintezaBannerView banner = new FintezaBannerView(context, ZONE_ID, WEBSITE_URL); |
In {WEBSITE_URL}, specify the application name or website domain the zone corresponds to. For {ZONE_ID}, indicate Finteza ad zone ID. These parameters are mandatory.
You can additionally set the rotation time in seconds by calling the banner.setRotationTime method. The default time is set to 10 minutes.
banner.setRotationTime(20); |
Loading content #
Call the load method to launch the content upload from Finteza into your banner.
When working via XML Layout, obtain the banner using the findViewById method by passing the banner ID from the android:id property into it. The call the load method for it.
FintezaBannerView banner = findViewById(R.id.finteza_banner);
|
If the banner is created programmatically, call load for it:
banner.load(); |
Additional settings #
You can specify callback to receive notifications about the content loading status. This can be done by implementing the OnBannerLoadListener interface:
banner.setOnLoadListener(new OnBannerLoadListener() {
|
Parameter overriding #
You can override the zone ID and the site address specified through XML Layout or in the FintezaBannerView constructor. This can be donne by calling the following methods:
banner.setZoneId(ZONE_ID); // zone ID
|
Ads rotation #
Ads rotation time is set by the rotationTime property in seconds (the default value is 10 minutes). For rotating banners, the load method should be called every time the parent view containing the banner is displayed. This does not result in unnecessary network requests until the time specified in rotationTime expires.
banner.rotationTime = 60 // rotation every minute |
To disable rotation, set rotationTime = 0.
The rotationTime parameter should be specified before calling the load method. |