Displaying ads
If your application displays ads, you can relocate the entire ad management to Finteza. Advertising campaigns on iOS devices can be launched just in time and for all users at once. No application modifying, waiting for the publication of a new version in App Store and updating all devices are required.
In fact, you only need to define ad areas in your application, while the entire contents management, detailed statistics and conversions are available in Finteza.
Although advertisements are called banners in Finteza, they are not limited to static images and GIF animations. A banner is a container that may include a variety of contents types, including HTML5: landing pages, carousels, pop-ups, etc.
The FintezaBannerView class is responsible for downloading and displaying the banner contents in SDK. It is derived from UIView making it possible to add it to the hierarchy of iOS application types in the standard way.
Creating an advertisement zone in Finteza #
Before creating a banner in the application, configure an advertisement zone in Finteza. It allows you to manage the ad block contents.
Go to the Websites section of the Finteza panel, find your application and click "new zone":
- Set the name and allowed content formats. JPG, PNG, GIF and HTML are currently supported.
- Set the zone size if known in advance or select automatic zone scaling.
- Save the changes and copy the zone ID. It should be specified in the application.
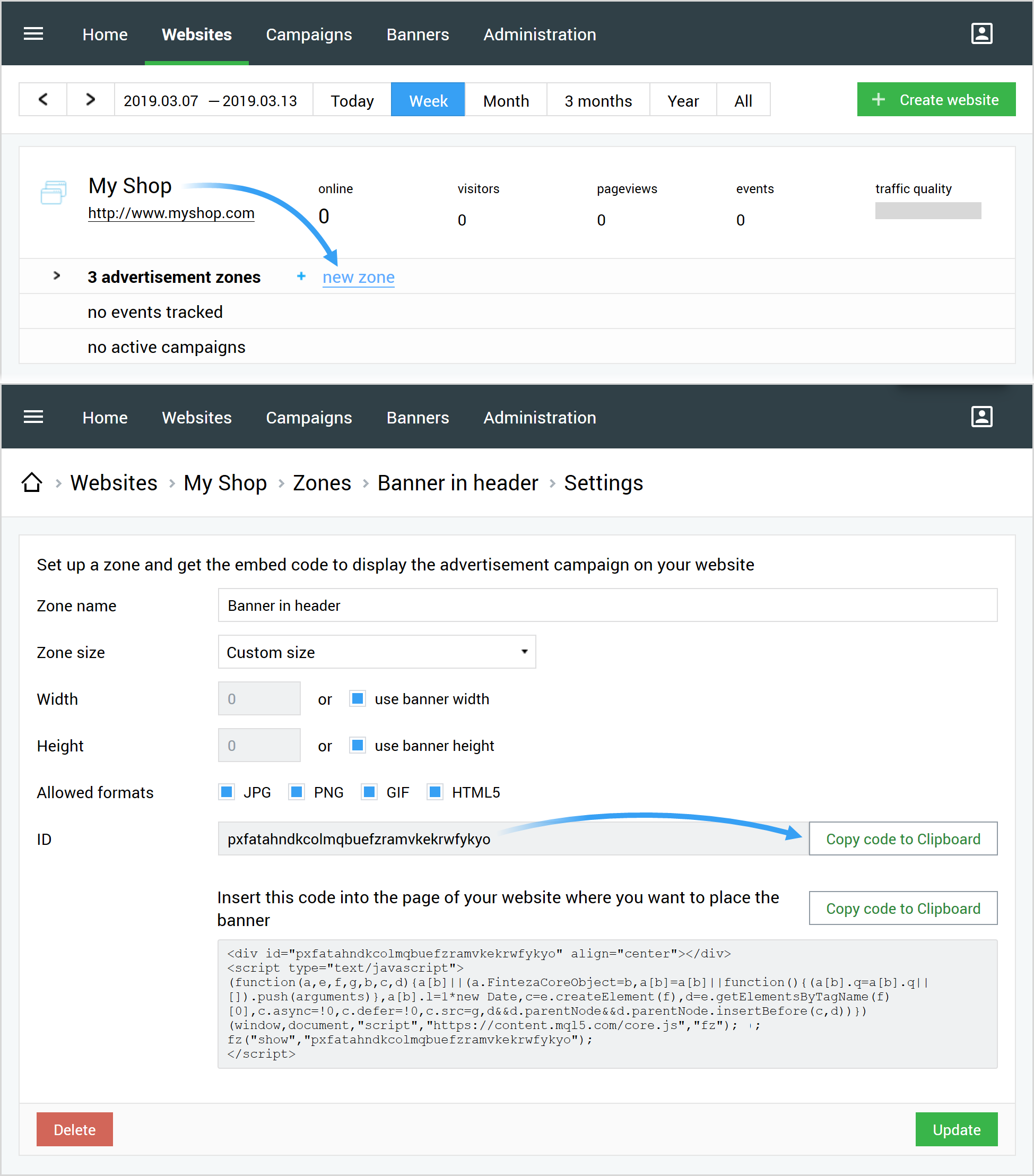
Creating a banner in the application #
You can create a banner via Interface Builder or programmatically.
Interface Builder
Create a new interface element of UIView type. Set FintezaBannerView in the Custom Class field of the "Identity inspector" tab. Set the necessary limitations for banner placement.
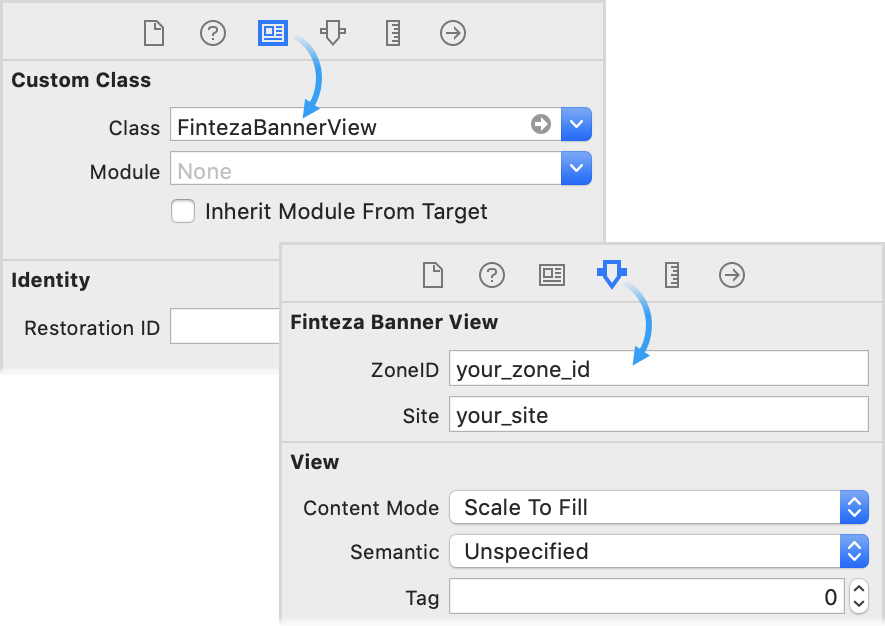
The banner size can be defined based on intrinsicContentSize. |
Programmatically
Use the following code to create a banner with certain dimensions:
Objective-C:
FintezaBannerView *banner = [[FintezaBannerView alloc] initWithFrame:bannerFrame]; |
Swift:
var banner = FintezaBannerView(frame: bannerFrame) |
Use the following code to create a banner occupying the entire view (using Auto Layout):
Objective-C:
FintezaBannerView *banner = [[FintezaBannerView alloc] initWithFrame:CGRectZero];
|
Swift:
let banner = FintezaBannerView(frame: .zero)
|
Initialization #
Set the zone ID and website address for the banner.
Objective-C and Swift:
banner.zoneID = @"{ZONE_ID}";
|
For the {ZONE_ID}, set Finteza ad zone ID. In {WEBSITE_URL}, set the application name or website domain the zone corresponds to.
These parameters can also be set in Interface Builder. To do this, select the FintezaBannerView class object and go to the "Attributes inspector" tab:
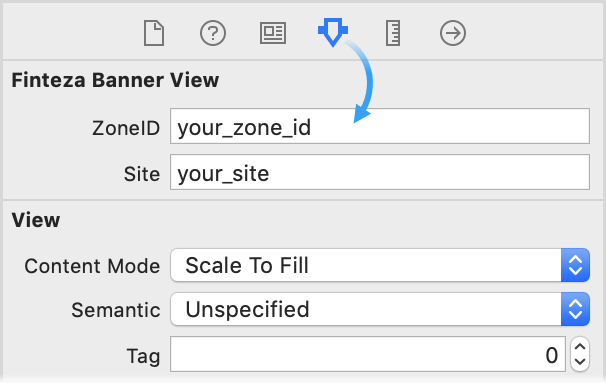
Downloading content #
Call the load method to launch the content download.
Objective-C:
[banner load]; |
Swift:
banner.load() |
You can specify a delegate object to receive notifications about the content download status. To do this, set an object implementing the protocol in the delegate property:
@protocol FintezaBannerViewDelegate <NSObject>
|
For example, a delegate allows adding a banner to the parent view only after the content is downloaded.
Defining the banner size based on intrinsicContentSize #
If you use Auto Layout, the banner size can be set based on the standard intrinsicContentSize property.
For FintezaBannerView, intrinsicContentSize is equal to the value set in the zone settings in Finteza. The size of the zone becomes known at the banner request stage.
If no exact zone size is specified ("Custom Size, use banner width\height" is set in the zone settings), intrinsicContentSize is defined based on the uploaded banner content. For JPG, PNG and GIF banners, this is the image size.
This method is not suitable for HTML and adaptive banners, since it is impossible to explicitly determine the content size for them. |
Ads rotation #
Ads rotation time is set by the rotationTime property in seconds (the default value is 10 minutes). For rotating banners, the load method should be called every time the parent view containing the banner is displayed (for example, in viewWillAppear). This does not result in unnecessary network requests until the time specified in rotationTime expires.
Objective-C and Swift:
banner.rotationTime = 60 // rotation every minute |
To disable rotation, set rotationTime = 0.
The rotationTime parameter should be specified before calling the load method. |